Vue Js Array.isArray Function : The isArray() method in JavaScript is used to determine whether a given object is an array or not. This method returns true if the object is an array; otherwise, it returns false. The method is a static property of the JavaScript Array object, which means it can only be accessed using the syntax “Array.isArray()”.
In Vue.js, you can use the isArray() method to check whether a data property is an array or not
Overall, the isArray() method is a simple yet useful function for checking whether an object is an array or not in Vue.js.
Check whether an element is in an array or not in Vue.js.
Vue.js provides the Array.isArray()
function to quickly determine if an object is an array or not. This can be used in the data()
method to define an array called country
. In the methods
section, a function called myFunction()
can be defined to set the result
variable to the value returned by the Array.isArray()
function called on the country
array.
Overall, this code snippet demonstrates how to use Array.isArray()
to check if an object is an array or not in Vue
Vue js Array.isArray Function | Example 1
<div id="app">
<button @click='myFunction' class='btn btn-primary'>check Array </button>
<p>{{result}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
country:['Australia','England','America','India','China','Russia'],
result : ''
}
},
methods:{
myFunction(){
this.result = Array.isArray(this.country);
},
}
}).mount('#app')
</script>
The above example returns true if an exist element is array else it will return false.
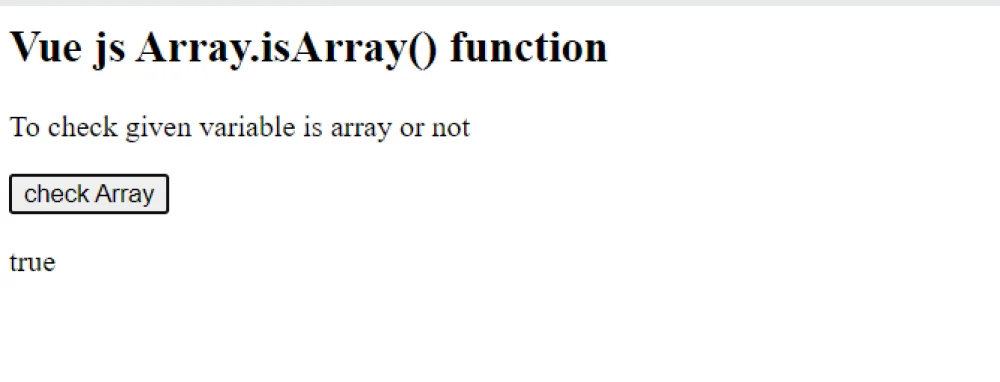
How to determine in Vue JS whether an object with data is in an array
To check if an object is an array in Vue.js, use Array.isArray(). It returns a boolean value indicating if the object is an array or not. For example, if you have an object called “country” and want to check if it’s an array, you can use Array.isArray(this.country) in your Vue.js methods.
Vue js Array.isArray method | Example 2
<div id="app">
<button @click='myFunction' class='btn btn-primary'>check Array </button>
<p>{{result}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
countries:[
{
'countryName':'Australia',
'capitalName':'Canberra'
},
{
'countryName':'England',
'capitalName':'London'
},
{
'countryName':'India',
'capitalName':'New Delhi'
},
],
result : ''
}
},
methods:{
myFunction(){
this.result = Array.isArray(this.countries);
},
}
}).mount('#app')
</script>
The above Example returns true if an object is array else it will return false
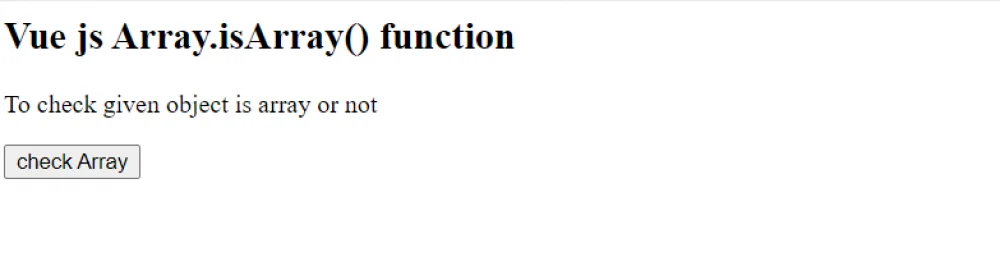